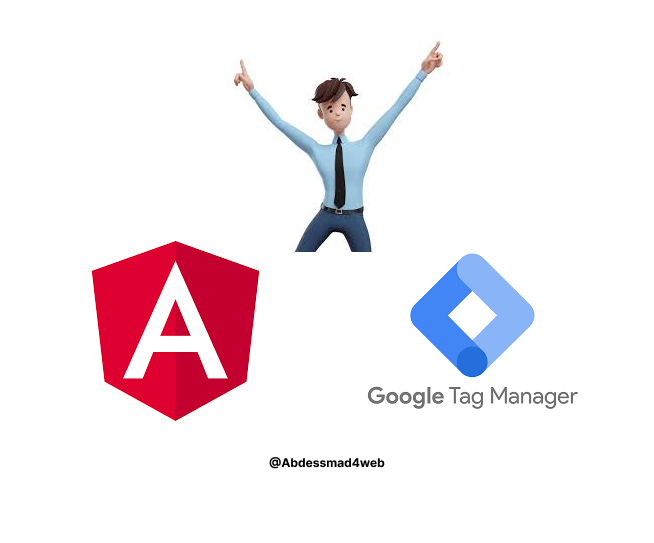
This guide offers a straightforward approach to integrating Google Tag Manager (GTM) into an Angular app to track page views and events. While there are libraries and modules available for this purpose, this method focuses on directly integrating the GTM tag and referencing it in the Angular app.
Integration Steps:
1. Integrate GTM code in `index.html:
— Obtain the GTM script when signing up for GTM.
— Place this script in the `<head>` tag of your Angular app’s `index.html` file.
Example:
<head>
<meta charset="utf-8">
<title>TestApp</title>
<base href="/">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="icon" type="image/x-icon" href="favicon.ico">
<! - Google Tag Manager →
<script>(function (w, d, s, l, i) {
w[l] = w[l] || []; w[l].push({
'gtm.start':
new Date().getTime(), event: 'gtm.js'
}); var f = d.getElementsByTagName(s)[0],
j = d.createElement(s), dl = l != 'dataLayer' ? '&l=' + l : ''; j.async = true; j.src =
'https://www.googletagmanager.com/gtm.js?id=' + i + dl; f.parentNode.insertBefore(j, f);
})(window, document, 'script', 'dataLayer', 'GTM-INSERT_YOUR_CODE_FROM_GTM_HERE');</script>
<! - End Google Tag Manager →
</head>
2. Ensure `window.dataLayer` is available:
— Initialize the `window.dataLayer` object to ensure it exists before GTM starts working.
Example:
<script>
window.dataLayer = window.dataLayer || [];
</script>
3. Create a window reference service (optional):
— Optionally, create a service to access the window object in different components.
4. Create a dataLayer service:
— Create a service to manage all dataLayer calls, keeping the dataLayer-related code in one place.
Example:
import {
Injectable
}
from '@angular/core';
import {
WindowReferenceService
}
from './window-reference.service';
@Injectable({
providedIn: 'root'
})
export class DataLayerService {
private window;
constructor(private windowRef: WindowReferenceService) {
this.window = windowRef.nativeWindow; // initialize the window to what we get from our window service
}
private pingHome(obj) {
if (obj) this.window.dataLayer.push(obj);
}
logPageView(url) {
const hit = {
event: 'content-view',
pageName: url
};
this.pingHome(hit);
}
logEvent(event, category, action, label) {
const hit = {
event: event,
category: category,
action: action,
label: label
};
this.pingHome(hit);
}
logCustomDimensionTest(value) {
const hit = {
event: 'custom-dimension',
value: value
};
this.pingHome(hit);
}
// Add more custom methods as needed by your app.
}
5. Track page views:
— Subscribe to router events and call the `logPageView` method of the `DataLayerService` to track page views.
Example:
import {
Router,
NavigationEnd
} from '@angular/router';
import {
DataLayerService
} from './data-layer.service';
export class AppComponent {
constructor(private _router: Router, private _dataLayerService: DataLayerService) {
this._router.events.subscribe(event => {
if (event instanceof NavigationEnd) {
this._dataLayerService.logPageView(event.url);
}
});
}
}
6. Track events or custom dimensions:
— Use the `logEvent` method in components where you need to track events.
Example:
import {
DataLayerService
} from './data-layer.service';
export class MyTestComponent {
constructor(private _dataLayerService: DataLayerService) {}
buttonOfInterestClicked() {
this._dataLayerService.logEvent('ButtonClicked', 'Buttons', 'Clicked', 'InterestingButton');
// Continue with the logic for what needs to be done in this method.
}
}
This approach ensures a streamlined integration of GTM into your Angular app, making it easier to manage and track various interactions.
If you found this information useful, please consider sharing the article with others.