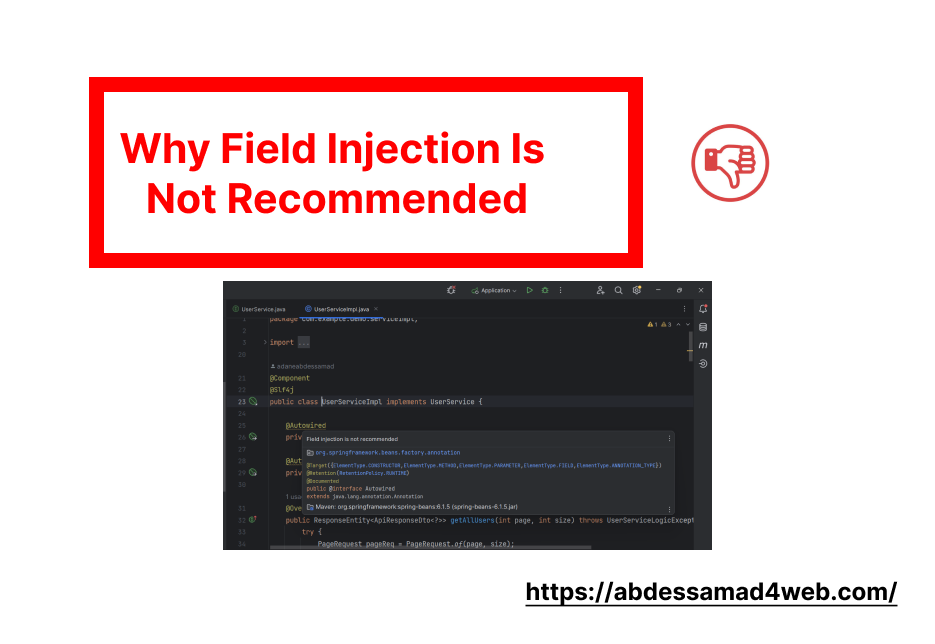
In Spring Boot, Dependency Injection (DI) is a powerful mechanism that simplifies how objects manage their dependencies. While Spring provides several ways to inject dependencies, including field injection, constructor injection, and setter injection, field injection is generally discouraged.
In this article, we’ll explore why field injection is not recommended in Spring Boot, focusing on the potential risks and alternative approaches to use.
1. What is Dependency Injection?
Dependency Injection (DI) is a design pattern where objects receive their dependencies from external sources, rather than creating them internally. This promotes loose coupling and enhances testability. In Spring Boot, dependencies can be injected in the following ways:
- Constructor Injection
- Setter Injection
- Field Injection
Field injection, though convenient and easy to implement, can lead to several issues.
2. Why Field Injection is Discouraged
Let’s take a closer look at the reasons field injection is not considered a best practice.
2.1. Null-Safety and Initialization Issues
One of the major problems with field injection is the risk of NullPointerException. With field injection, there’s no guarantee that dependencies will be properly initialized before the class is used.
@Service
public class TeacherService {
@Autowired
private UserService userService;
public void teach() {
userService.doSomething(); // This can throw NullPointerException if not initialized
}
}
If the TeacherService
is instantiated without the Spring context, userService
might not be initialized, leading to a NullPointerException
.
To avoid this, constructor injection can be used, ensuring that dependencies are injected when the object is created:
@Service
public class TeacherService {
private final UserService userService;
public TeacherService(UserService userService) {
this.userService = userService;
}
public void teach() {
userService.doSomething(); // Safe: userService is guaranteed to be initialized
}
}
With constructor injection, Spring ensures that the required dependencies are passed to the constructor during object creation. This makes the TeacherService
null-safe and prevents accidental misuse.
2.2. Immutability
Using field injection makes it difficult to create immutable classes. When a class is immutable, its state cannot be changed after it is created, which is a good design practice in many cases. Field injection does not allow for final
fields because fields are not initialized through constructors. This prevents immutability.
Let’s say you want the UserService
dependency in the TeacherService
to be immutable:
javaCopy code@Service
public class TeacherService {
private final UserService userService;
public TeacherService(UserService userService) {
this.userService = userService; // Dependency is immutable
}
}
By using constructor injection, the UserService
can be final
, ensuring it cannot be changed after the object is constructed. Field injection doesn’t offer this flexibility, which could lead to mutable dependencies and unintended side effects.
2.3. Violation of Single Responsibility Principle
Field injection can also contribute to violating the Single Responsibility Principle (SRP). If too many dependencies are injected via fields, the class might end up doing more than it should.
For instance:
javaCopy code@Service
public class TeacherService {
@Autowired
private UserService userService;
@Autowired
private ClassRoomService classRoomService;
@Autowired
private StudentService studentService;
public void manageClass() {
// Manage users, classrooms, students - multiple responsibilities
}
}
In contrast, constructor injection often forces you to think about whether the class is becoming too complex. If a constructor requires too many parameters, it’s a sign that the class may be taking on too many responsibilities and should be refactored.
2.4. Difficulty in Unit Testing
Field injection makes unit testing more difficult. When testing a class with field-injected dependencies, you may need to use reflection or mock injection frameworks to manually inject dependencies into private fields.
Here’s a problematic scenario with field injection:
javaCopy code@Test
public void testTeacherService() {
TeacherService teacherService = new TeacherService();
// Now we have to manually inject userService, which is cumbersome
}
With constructor injection, you can simply pass the mock objects as dependencies during testing:
javaCopy code@Test
public void testTeacherService() {
UserService mockUserService = Mockito.mock(UserService.class);
TeacherService teacherService = new TeacherService(mockUserService);
// Now teacherService is ready to use in tests
}
This approach makes unit testing much more straightforward and avoids the complexity of injecting dependencies through reflection.
2.5. Circular Dependencies
Field injection can allow circular dependencies to go unnoticed until runtime. Circular dependencies occur when two or more classes depend on each other, leading to errors during object creation.
For instance:
javaCopy code@Component
public class TeacherService {
@Autowired
private StudentService studentService;
}
@Component
public class StudentService {
@Autowired
private TeacherService teacherService;
}
With constructor injection, such issues are caught at compile time because the constructors would not be able to resolve the dependencies:
javaCopy code@Component
public class TeacherService {
private final StudentService studentService;
public TeacherService(StudentService studentService) {
this.studentService = studentService;
}
}
3. Alternatives to Field Injection
Now that we’ve outlined the problems with field injection, let’s explore the two better alternatives:
3.1. Constructor Injection
Constructor injection is the preferred way of injecting dependencies in Spring Boot. It guarantees immutability and helps prevent null references.
javaCopy code@Service
public class TeacherService {
private final UserService userService;
public TeacherService(UserService userService) {
this.userService = userService;
}
}
3.2. Setter Injection
For optional dependencies, setter injection can be a good option. Setter injection allows you to inject dependencies when necessary without making them mandatory.
javaCopy code@Service
public class TeacherService {
private UserService userService;
@Autowired
public void setUserService(UserService userService) {
this.userService = userService;
}
}
4. Conclusion
Field injection might seem convenient, but it can lead to several design and runtime issues. Constructor injection is usually the best practice for injecting required dependencies in Spring Boot, as it promotes immutability, prevents null-related issues, and enhances testability. Setter injection, on the other hand, is useful for optional dependencies. By adopting constructor or setter injection, you can avoid the pitfalls of field injection and build more robust, maintainable Spring applications.